Test Automation using Sikuli
Why Sikuli is used in Selenium automation?
Sikuli automates anything you see on screen using the image recognition method to identify GUI elements. Sikuli script allows users to automate GUI interaction by using screenshots.
Procedures to integrate Silkuli with Selenium:-
- Download sikuli-java.jar from https://launchpad.net/sikuli/+download
- Double click on sikuli-java.jar. One popup window will appear
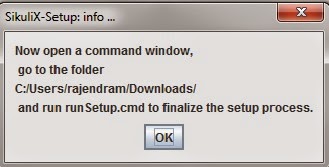
- Click on 'OK' button.Now you can see one command prompt file named by runSetup.cmd
- Double click on runSetup.cmd.
- The command prompt file will be executed and one popup window will appear.
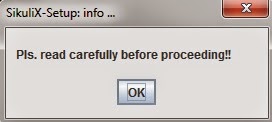
- Click on 'OK' button. Another popup window will appear.
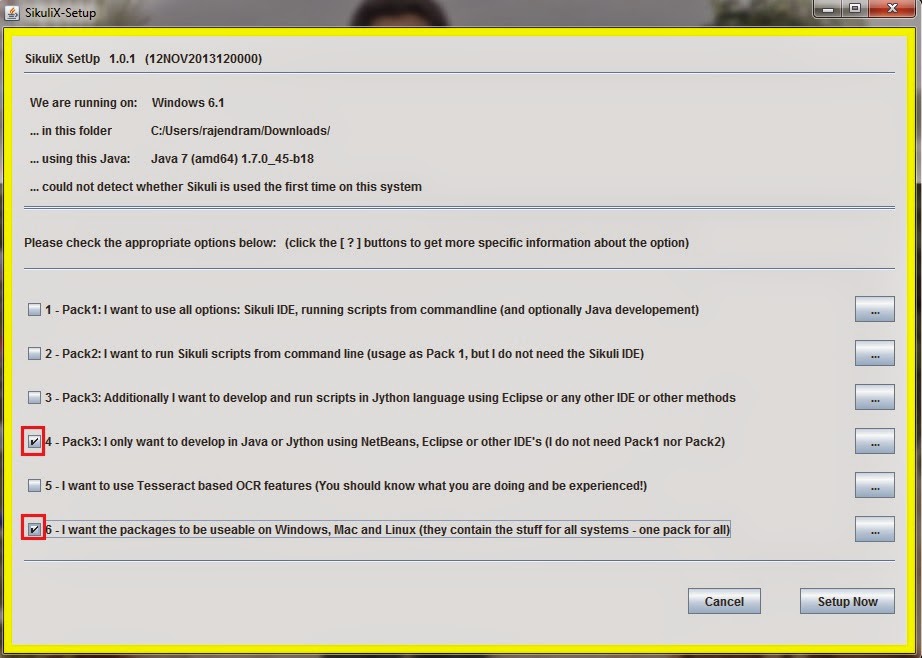
- select 4th and 6th check boxes and click on 'Setup Now' button.One popup window will appear.
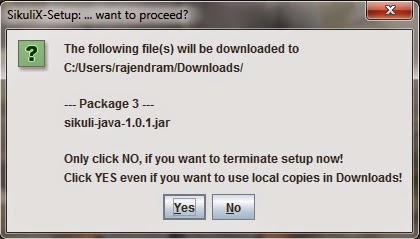
- Click on 'Yes' button. It will create sikuli-java.jar.
- You have to add this sikuli-java.jar to 'Referenced Libraries' of the Project.
Steps to Create Sikuli Java Project:
Step #1: Open Eclipse.
Step #2: Create a java project File -> New -> Java Project
Step #3:
- Right Click on the project
- Go to Build path -> Configure Build Path
- Switch to Libraries tab
- Click “Add External Jars” button and Add sikuli-java.jar in the Build path.
- Click “Ok”
(Click on image to enlarge)
sikuli-java.jar , will be added to your project build path. You’re done. Now you can start writing Sikuli scripts inside this project.
Some Sikuli Methods:
#1: Creating Object for Screen Class
Screen is a base class provided by Sikuli. We need to create object for this screen class first, then only we can access all the methods provided by Sikuli.
Screen is a base class provided by Sikuli. We need to create object for this screen class first, then only we can access all the methods provided by Sikuli.
Syntax:
Screen s=new Screen();
Screen s=new Screen();
#2: Click On An Element
This method used to Click on the specific image present on the screen.
This method used to Click on the specific image present on the screen.
Syntax:
s.click(“<<image name>>”);
s.click(“<<image name>>”);
Example:
s.click(“test.png”);
s.click(“test.png”);
#3: Right Click On An Element
This method used to right click on the specific image present on the screen.
This method used to right click on the specific image present on the screen.
Syntax:
s.rightClick(“<<image name>>”);
s.rightClick(“<<image name>>”);
Example:
s.rightClick(“test.png”);
s.rightClick(“test.png”);
#4: find An Element
This method used to find a specific element present on the screen.
This method used to find a specific element present on the screen.
Syntax:
s.find(“<<image name>>”);
s.find(“<<image name>>”);
Example:
s.find(“test.png”);
s.find(“test.png”);
#5: Double Click on An Element
This method used to trigger double click event on a specific image present on the screen.
This method used to trigger double click event on a specific image present on the screen.
Syntax:
s.doubleClick(“<<image name>>”);
s.doubleClick(“<<image name>>”);
Example:
s.doubleClick(“test.png”);
s.doubleClick(“test.png”);
#6: Check whether an Element present on the Screen
This Method is used to check whether the specified element is present on the screen.
This Method is used to check whether the specified element is present on the screen.
Syntax:
s.exists(“<<image name>>”);
s.exists(“<<image name>>”);
Example:
s.exists(“test.png”);
s.exists(“test.png”);
#7: Type a string on a Textbox
This Method is used to enter the specified text on the Text box.
This Method is used to enter the specified text on the Text box.
Syntax:
s.type(“<<image name>>”,”String to be typed”);
s.type(“<<image name>>”,”String to be typed”);
Example:
s.type(“test.png”,”HI!!”);
s.type(“test.png”,”HI!!”);
#8: Wheeling on a particular image
This method is used to perform wheeling action on the element image.
This method is used to perform wheeling action on the element image.
Syntax:
s.wheel(“<<image name>>”,<<int position>>,<<int direction>>);
s.wheel(“<<image name>>”,<<int position>>,<<int direction>>);
Example:
s.wheel(“test.png”,25,0);
s.wheel(“test.png”,25,0);
#9: Drag and Drop a Image/ElementThis method is used to drag and drop a specified image from source position to target position.
Syntax:
s.dragDrop(“<<source image name>>”,”<<target image name>>”);
s.dragDrop(“<<source image name>>”,”<<target image name>>”);
Example:
s.dragDrop(“test.png”,”test1.png”);
s.dragDrop(“test.png”,”test1.png”);
#10: Roll Hover on a particular image
This method is used to perform roll hover event on the specified image.
This method is used to perform roll hover event on the specified image.
Syntax:
s.hover(“<<image name>>”);
s.hover(“<<image name>>”);
Example:
s.hover(“test.png”);
s.hover(“test.png”);
#11: Paste Copied String
This method used to paste text on the specified textbox.
This method used to paste text on the specified textbox.
Syntax:
s.paste(“<<image name>>”,”test”);
s.paste(“<<image name>>”,”test”);
Example:
Let's automate a simple scenario
(File Upload Using sikuli)
Steps :-
- Navigate to http://sandbox.checklist.com/login
- Enter UserName and Password and click on 'Login' button
- Click on 'checklist1' link
- Click on 'upload' link which will open a window to choose the file. As it is window based we need to choose and upload file using sikuli.
Please follow the below mentioned program to upload file using sikuli :-
package pkg_selenium;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.sikuli.script.Screen;
public class FileUploadUsingSikuli {
public static WebDriver driver;
public static void main(String[] args) throws InterruptedException {
// TODO Auto-generated method stub
String userPath = System.getProperty("user.dir");
System.out.println(userPath);
// Initialize WebDriver
driver = new FirefoxDriver();
// Wait For Page To Load
driver.manage().timeouts().pageLoadTimeout(60, TimeUnit.SECONDS);
// Go to URL
driver.get("http://sandbox.checklist.com/login");
// Maximize Window
driver.manage().window().maximize();
// Enter UserName
driver.findElement(By.name("j_username")).clear();
driver.findElement(By.name("j_username")).sendKeys("rajendra1988@gmail.com");
// Enter Password
driver.findElement(By.name("j_password")).clear();
driver.findElement(By.name("j_password")).sendKeys("test123");
// Click on 'Login' button
driver.findElement(By.name("login")).click();
Thread.sleep(15000L);
// Click on 'checklist1' link
driver.findElement(By.xpath("//*[@id='userChecklists']/li")).click();
Thread.sleep(5000L);
// Click on 'upload' link
driver.findElement(By.id("taskUploadFile")).click();
Thread.sleep(5000L);
// Initialize Screen Object
Screen scr = new Screen();
// Select Files
try{
scr.type(userPath+"\\img\\FileUpload.png",userPath+"\\Repository\\MouseOverScript.txt");
// Click on 'Open' button
scr.click(userPath+"\\img\\Open.png");
}catch(Throwable t){
System.out.println("Exeception came while selecting File :"+t.getMessage());
}
System.out.println("Test Completed");
Thread.sleep(30000L);
driver.close();
}
}
Hello,
ReplyDeleteThe Article on Test Automation using Sikuli is nice. It give detail information about it .Thanks for Sharing the information about Test Automation. Software Testing Services
Thank You...
ReplyDelete